반응형
안녕하세요. PSYda입니다.
이번 포스팅에서는 Pandas DataFrame의 통계에 대해 알아보겠습니다.
소개할 내용은 아래와 같습니다.
- DataFrame의 통계 함수 소개(주요통계량 출력, 평균, 표준편차, 최대/최소값, 상관계수 등)
- DataFame의 통계에 대한 시각화(히스토그램, 상관관계)
실습에 사용한 데이터는 fifa19에 등장하는 선술들의 능력치 데이터 입니다.
아래에서 다운로드 받을 수 있습니다.
Ch6_1_fifaStats.csv
다운로드
6.Pandas DataFrame 통계
DataFrame의 주요한 통계 함수는 아래와 같습니다.
- Describe : 전체 주요 통계량 출력
- sum : 합계 출력
- cumsum : 누적합계 출력
- mean : 평균값 출력
- Std : 표준 편차 출력
- var : 분산 출력
- median : 중앙값 출력
- count : 데이터 개수 출력
- min, max, idxmin, idxmax : 최소값,최대값,최소값의 index, 최대값의 index 출력
- corr, cov : 상관계수, 공분산 출력
위의 통계 함수를 비롯한 대부분의 함수에서 axis 옵션을 이용해 사용할 축을 설정할 수 있습니다.
- axis = 0 : 열방향으로 통계량 산출(Default)
- axis = 1 : 행방향으로 통계량 산출
실습을 위해 Fifa19 선수들의 Stat 정보를 Load
import pandas as pd
from pandas import Series, DataFrame
import numpy as np
players = pd.read_csv('data/Ch6_1_fifaStats.csv', index_col = 'Name')
players.head()
ShortPassing | Dribbling | LongPassing | BallControl | |
---|---|---|---|---|
Name | ||||
L. Messi | 90.0 | 97.0 | 87.0 | 96.0 |
Cristiano Ronaldo | 81.0 | 88.0 | 77.0 | 94.0 |
Neymar Jr | 84.0 | 96.0 | 78.0 | 95.0 |
De Gea | 50.0 | 18.0 | 51.0 | 42.0 |
K. De Bruyne | 92.0 | 86.0 | 91.0 | 91.0 |
6.1 전체 통계량 요약 보기(describe)
describe 함수 : 컬럼별 전체 통계량을 요약해서 볼 수 있음
- 데이터 개수, 평균, 표준편차, 최소값, 4분위값, 최대값 출력
- 숫자형 컬럼만 통계량을 출력함
decribe 함수 호출
players.describe()
ShortPassing | Dribbling | LongPassing | BallControl | |
---|---|---|---|---|
count | 18159.000000 | 18159.000000 | 18159.000000 | 18159.000000 |
mean | 58.686712 | 55.371001 | 52.711933 | 58.369459 |
std | 14.699495 | 18.910371 | 15.327870 | 16.686595 |
min | 7.000000 | 4.000000 | 9.000000 | 5.000000 |
25% | 54.000000 | 49.000000 | 43.000000 | 54.000000 |
50% | 62.000000 | 61.000000 | 56.000000 | 63.000000 |
75% | 68.000000 | 68.000000 | 64.000000 | 69.000000 |
max | 93.000000 | 97.000000 | 93.000000 | 96.000000 |
6.2 함수별 통계량 보기
6.2.1 sum():합계 출력
players.sum()
ShortPassing 1065692.0
Dribbling 1005482.0
LongPassing 957196.0
BallControl 1059931.0
dtype: float64
6.2.2 cumsum():누적합계 출력
# 선수별 능력치 누적 합계 출력
players.head().cumsum(axis = 1)
ShortPassing | Dribbling | LongPassing | BallControl | |
---|---|---|---|---|
Name | ||||
L. Messi | 90.0 | 187.0 | 274.0 | 370.0 |
Cristiano Ronaldo | 81.0 | 169.0 | 246.0 | 340.0 |
Neymar Jr | 84.0 | 180.0 | 258.0 | 353.0 |
De Gea | 50.0 | 68.0 | 119.0 | 161.0 |
K. De Bruyne | 92.0 | 178.0 | 269.0 | 360.0 |
6.2.3 mean(): 평균값 출력
Stat별 평균(컬럼 기준)
players.mean()
ShortPassing 58.686712
Dribbling 55.371001
LongPassing 52.711933
BallControl 58.369459
dtype: float64
선수별 평균(행 기준)
players.mean(axis=1).head()
Name
L. Messi 92.50
Cristiano Ronaldo 85.00
Neymar Jr 88.25
De Gea 40.25
K. De Bruyne 90.00
dtype: float64
6.2.4 std(): 표준편차 출력
players.std()
ShortPassing 14.699495
Dribbling 18.910371
LongPassing 15.327870
BallControl 16.686595
dtype: float64
6.2.5 var():분산 출력
players.var()
ShortPassing 216.075157
Dribbling 357.602135
LongPassing 234.943613
BallControl 278.442466
dtype: float64
```text
### 6.2.6 median():중앙값 출력
```python
players.median()
ShortPassing 62.0
Dribbling 61.0
LongPassing 56.0
BallControl 63.0
dtype: float64
6.2.7 count() : 데이터 개수 출력
players.count()
ShortPassing 18159
Dribbling 18159
LongPassing 18159
BallControl 18159
dtype: int64
6.2.8 max(), min() : 최대값, 최소값 출력
players.max()
ShortPassing 93.0
Dribbling 97.0
LongPassing 93.0
BallControl 96.0
dtype: float64
players.min()
ShortPassing 7.0
Dribbling 4.0
LongPassing 9.0
BallControl 5.0
dtype: float64
6.2.9 idxmin(), idxmax():최대값,최소값에 해당하는 인덱스 출력
선수별 가장 낮은 능력치는?
players.idxmin(axis = 1).head()
Name
L. Messi LongPassing
Cristiano Ronaldo LongPassing
Neymar Jr LongPassing
De Gea Dribbling
K. De Bruyne Dribbling
dtype: object
스탯별 최고의 선수는?
players.idxmax()
ShortPassing L. Modrić
Dribbling L. Messi
LongPassing T. Kroos
BallControl L. Messi
dtype: object
6.2.10 corr(), cov(): 상관계수, 공분산 출력
전체 컬럼별 상관계수 행렬 출력
corr_mat = players.corr()
corr_mat
ShortPassing | Dribbling | LongPassing | BallControl | |
---|---|---|---|---|
ShortPassing | 1.000000 | 0.843722 | 0.895722 | 0.911451 |
Dribbling | 0.843722 | 1.000000 | 0.722465 | 0.938942 |
LongPassing | 0.895722 | 0.722465 | 1.000000 | 0.788650 |
BallControl | 0.911451 | 0.938942 | 0.788650 | 1.000000 |
특정 컬럼과 상관도가 높은 순서대로 출력
corr_mat['ShortPassing'].sort_values(ascending = False)
ShortPassing 1.000000
BallControl 0.911451
LongPassing 0.895722
Dribbling 0.843722
Name: ShortPassing, dtype: float64
전체 컬럼별 공분산 행렬 출력
cov_mat = players.cov()
cov_mat
ShortPassing | Dribbling | LongPassing | BallControl | |
---|---|---|---|---|
ShortPassing | 216.075157 | 234.531811 | 201.816840 | 223.564867 |
Dribbling | 234.531811 | 357.602135 | 209.410657 | 296.282958 |
LongPassing | 201.816840 | 209.410657 | 234.943613 | 201.712999 |
BallControl | 223.564867 | 296.282958 | 201.712999 | 278.442466 |
특정 컬럼과 공분산이 낮은 순서대로 출력
cov_mat['Dribbling'].sort_values(ascending = True)
LongPassing 209.410657
ShortPassing 234.531811
BallControl 296.282958
Dribbling 357.602135
Name: Dribbling, dtype: float64
6.3 통계량 시각화하기
6.3.1 histogram 시각화
players.hist(figsize = (15,10))
array([[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6CA2E6A0>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6CA2D898>],
[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6C860080>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6C8872E8>]],
dtype=object)
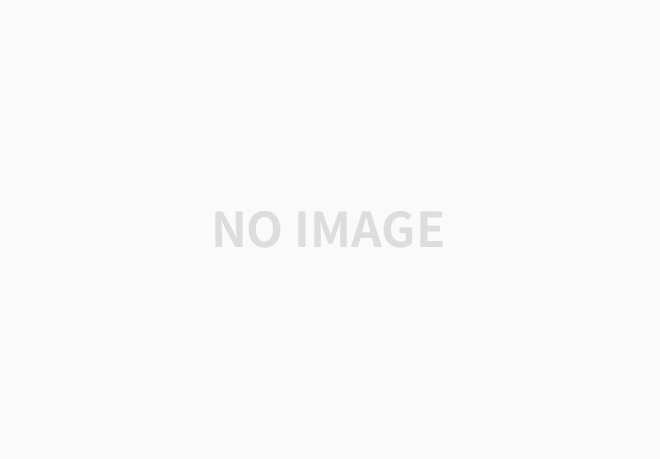
6.3.2 상관계수 그래프 시각화
pd.plotting.scatter_matrix(players, figsize = (15,10))
array([[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E38F1D0>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E6F50B8>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E3D6358>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E3FD898>],
[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E426E10>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E4573C8>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E47F940>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E4A8EF0>],
[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E4A8F28>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E4FE9E8>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E525F60>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E556518>],
[<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E580A90>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E5B2048>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E5D95C0>,
<matplotlib.axes._subplots.AxesSubplot object at 0x0000020A6E601B38>]],
dtype=object)
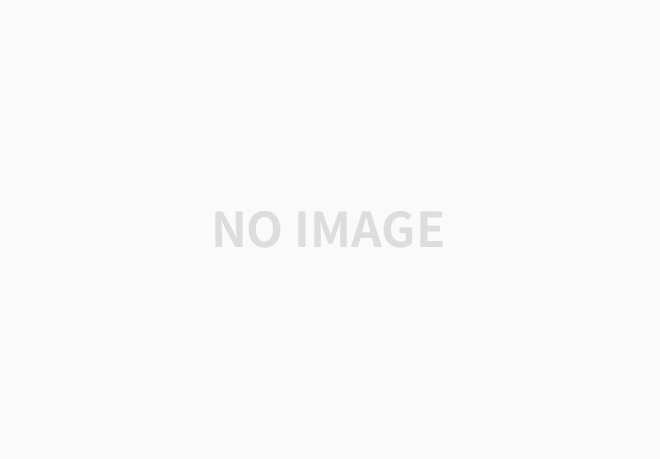
위의 Jupyter notebook 내용은 여기 Github에서도 확인 할 수 있습니다.
감사합니다.
반응형
'데이터 분석 > Pandas' 카테고리의 다른 글
[Pandas 기초]9.Series와 DataFrame에 함수 적용하기 (0) | 2019.08.29 |
---|---|
[Pandas 기초]8.Pandas DataFrame의 sort, rank함수 (0) | 2019.08.28 |
[Pandas 기초]6.Pandas DataFrame 산술 연산 (2) | 2019.08.22 |
[Pandas 기초]5.Pandas 데이터 파일 입출력 (3) | 2019.08.20 |
[Pandas 기초]4.여러 DataFrame 연결하기(Join) (0) | 2019.08.17 |